Top 5 React front-end Questions To Practice Before A Technical Interview Round
28th Jun 2022
Manu Arora
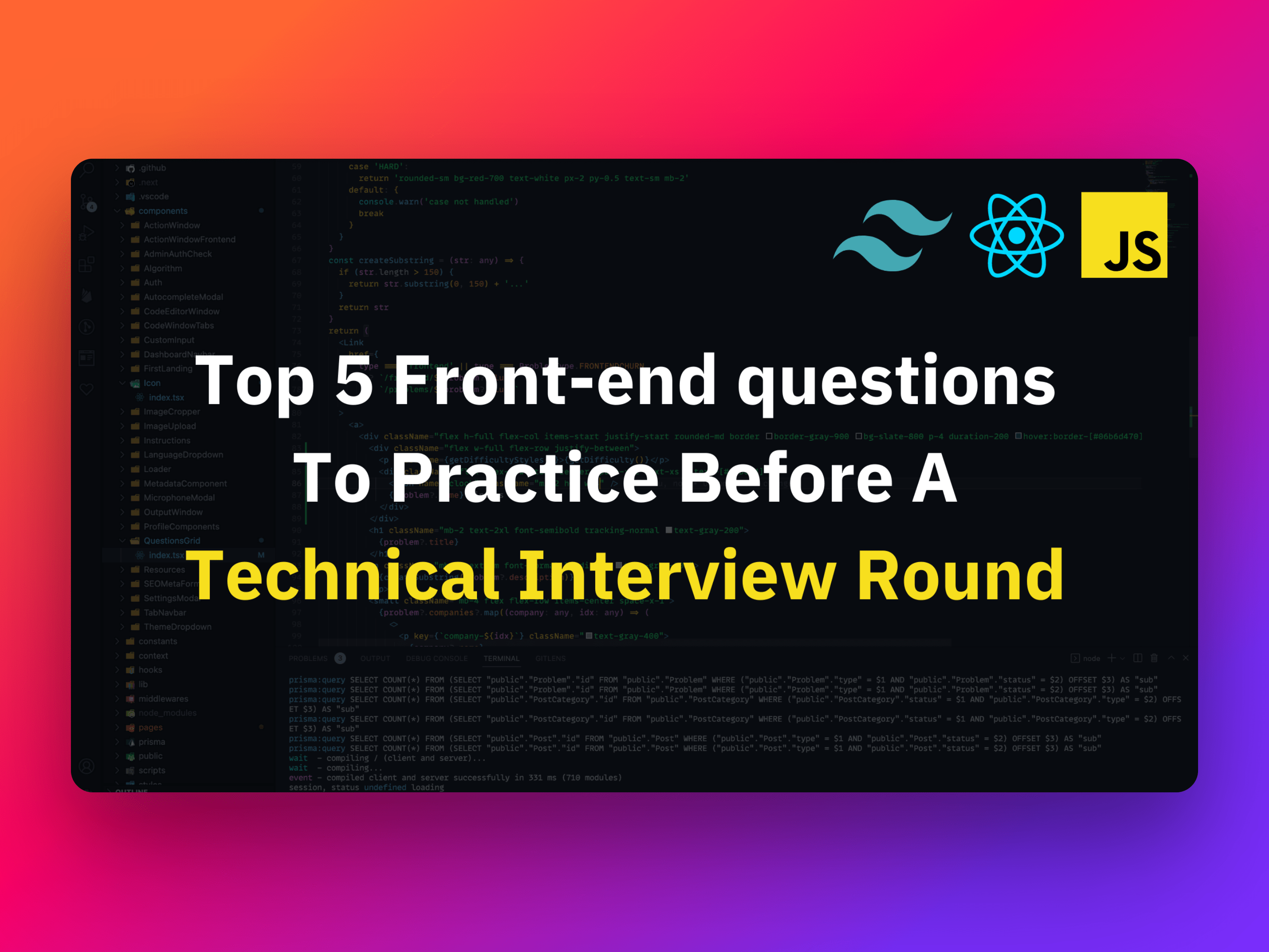
Technical Interviews can be tricky at times. They tend to test developer's everyday skills and assess how well versed they are with the everyday concepts that they use.
Not only this, preparing for a tech interview can be challenging because this requires patience and a bank of questions to practice from.
Today, we are going to discuss top 5 front-end interview questions asked in a technical interview round.
1. Debouncing
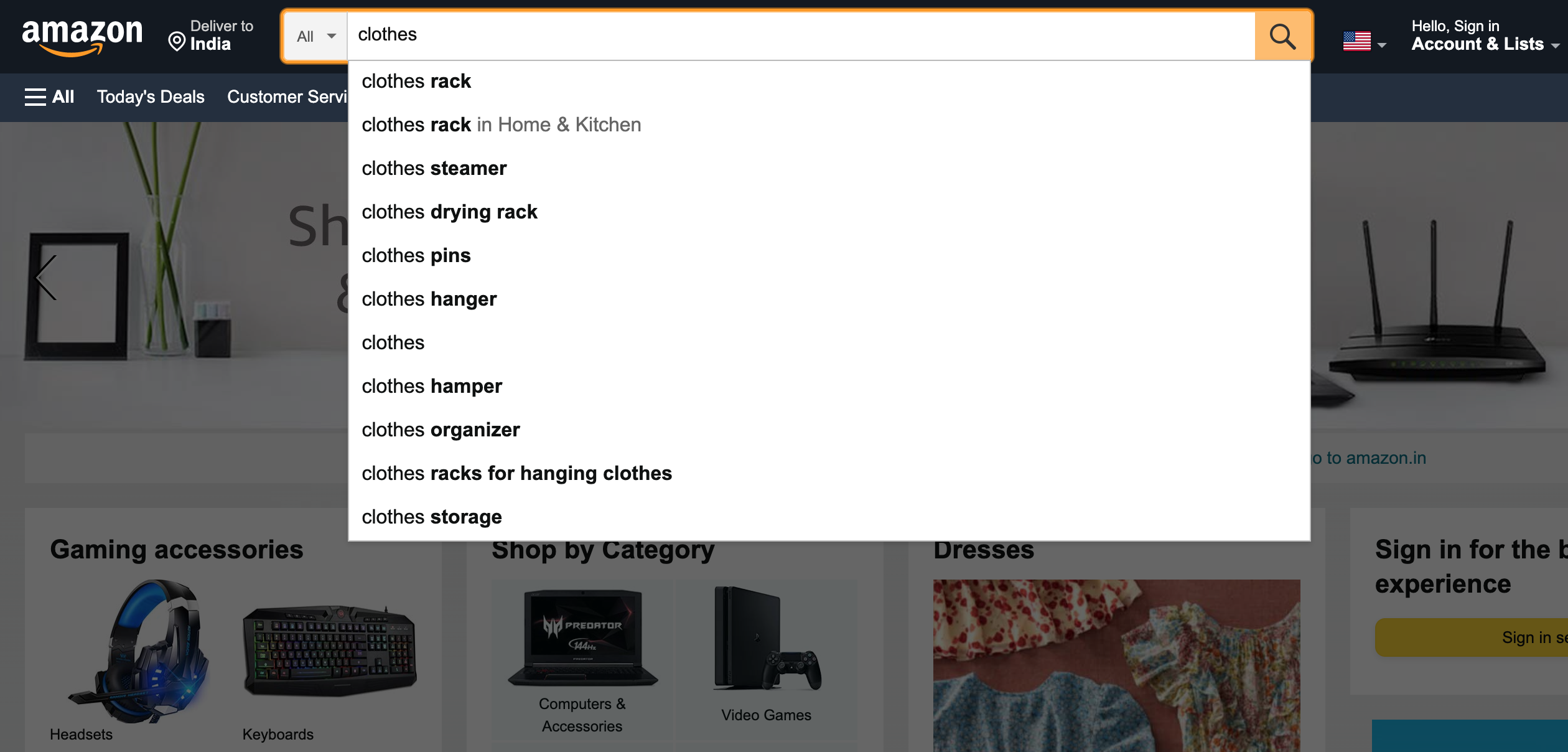
Consider the search bar at Amazon. It does the following things:
- When the user types, it DOES NOT immediately call the Backend API to fetch results.
- The API takes some time, the time when the user stops typing, and after that time has passed - the API is called and the results are populated.
In theory, Debouncing
is a method of preventing a function from being invoked too often, and instead of waiting a certain amount of time until it was last called before invoking it. A great example of where this is useful is an auto-completing input field that calls an API with the partial input to get a list of possible complete inputs.
Concepts tested:
- Interacting with Web APIs like
setTimeout()
andsetInterval()
- Knowledge of states and state management -
useState()
knowledge. - Knowledge of interacting with APIs with
axios
andfetch
methods.
Practice Debouncing at Algochurn
2. Feedback Stars
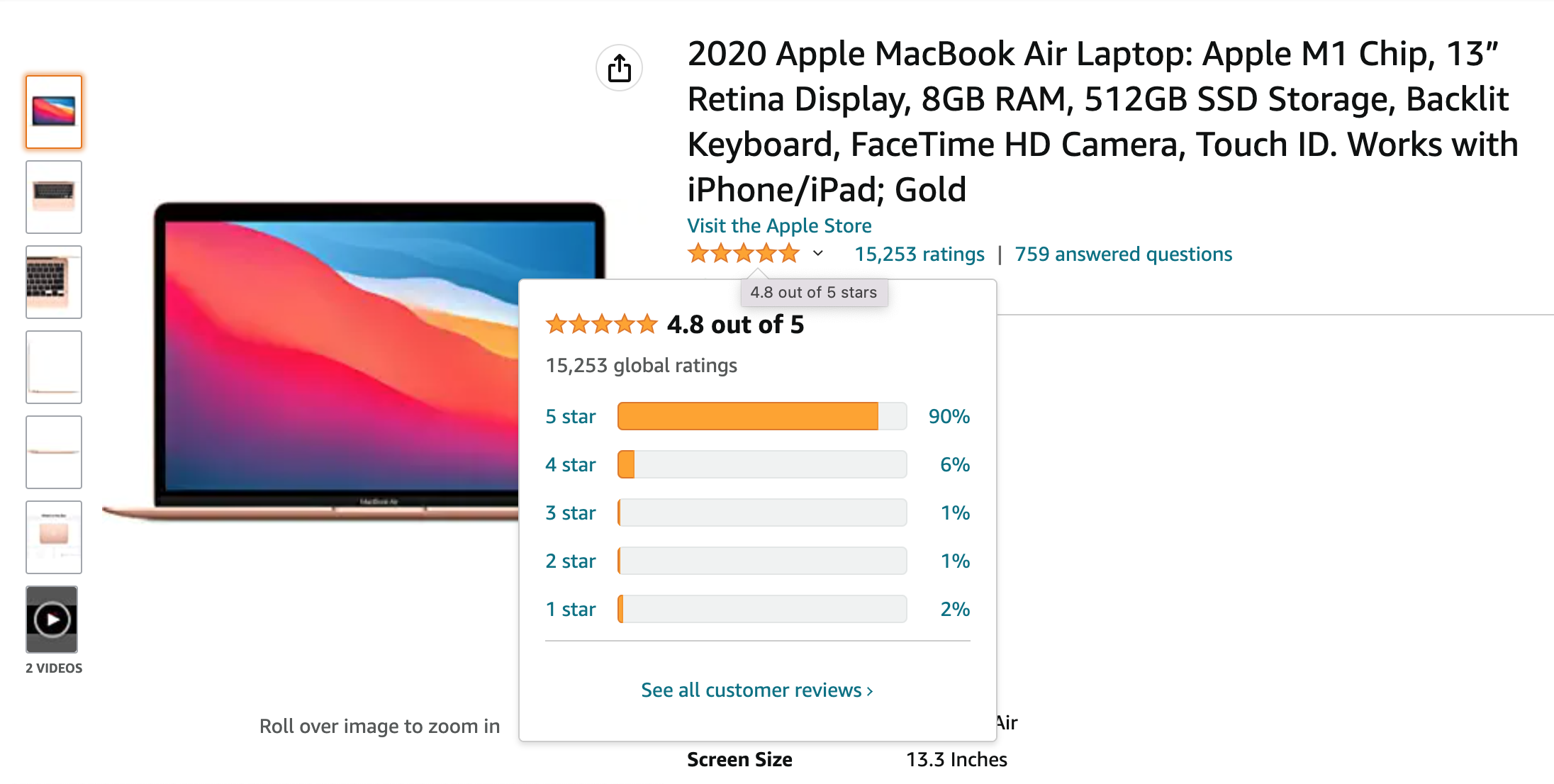
A popular question asked in Amazon
interview rounds, Feedback stars is the feedback component that takes in a numberOfStars
prop and renders the stars on the screen.
The star, when hovered over, highlights all the stars uptill that point.
Once the user clicks on any of the star, the ratings are generated and can be stored in a state variable, denoting the current rating assigned to the component.
This problem can seem a little simple on the surface but it is quite tricky once the candidate starts implementing them, especially in a time-based interview.
Concepts tested
- Handling
events
in Javascript and React - Event Delegation in Javascript
- State management using
useState()
- How to create a component in such a way that it takes in an Input and returns an output with the
change handlers
provided by the parent component.
Practice Feedback Stars at Algochurn
3. Folder Structure
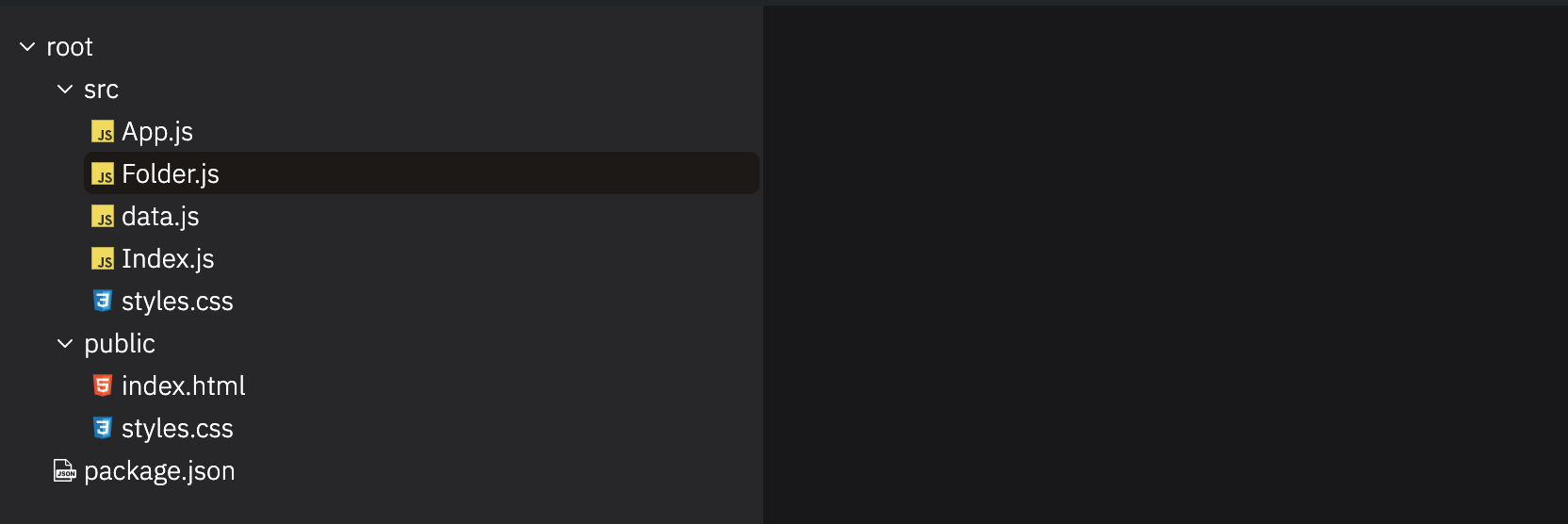
The window pane on the left hand side of your VS Code editor has a file explorer. Once you click on any folder, the sub folders open up and you can navigate to any file of your choice. This is called a basic folder structure setup.
Folder structure can be tricky since it involves recursion. But if you look at the problem itself, it is simple in terms of implementation. You just need to focus on the base case and the type of the data object that you're given.
Concepts tested:
- Recursion. One can use recursion to render the same component again and again if the current object has children.
- State management with
useState()
. - Performance optimizations and how the candidate handles them.
Practice Folder Strucure at Algochurn
4. Polyfills
A polyfill is a code snippet (in terms of javascript web architecture) used for modern world functionalities on older browsers that do not implement it natively. They're essential because it teaches various deep-level concepts of javascript. Here, we are going to implement map, filter, reduce and promise.all polyfills.
Essentially, A polyfill is a custom implementation of native javascript methods. Some of the popular javascript methods are:
map()
- Iterate over an array and return a resulting array.filter()
- Iterate over an array and return a resulting array based on a boolean.reduce()
- Iterate over an array and return a single value.forEach()
- Iterate over an arraypromise.all()
- Take in an array ofpromises
and resolve only when all of the promises in the array are resolved, otherwise reject.
Concepts tested:
- Javascript basics and fundamentals.
Callbacks
call()
,apply()
andbind()
methods- Promises
Practice Polyfills at Algochurn
5. Memoization
Memoization is an optimization technique used primarily to speed up computer programs by storing the results of expensive function calls and returning the cached result when the same inputs occur again.
In other terms, Memoization is a way for a function to essentially remember
the inputs value's processed results - so that the next the the same input parameters hit the function, it can return the results from the cache instead of again computing and consuming CPU memory.
Memoization is the single most question asked by almost all of the Big Tech companies out there. It is a real world concepts which are being used in most of the heavy loading websites today.
Concepts tested:
- Closures
- Javascript fundamentals and basics
- Promises
- Native Javascript functions understanding.
call()
,apply()
andbind()
Practice Memoization at Algochurn
Conclusion
These are the top questions that are being asked by tech companies at scale today - They primarily want to test you on your Javascript and React Fundamentals.
If you're a front-end engineer trying to clear coding and technical interviews, practice these problems beforehand to gain confidence and clear interviews with ease.
To ease out your preparation process, visit Algochurn - Practice real-world problems in a real world environment without hassle.