Junior front end developer interview questions
9th Oct 2022
Manu Arora
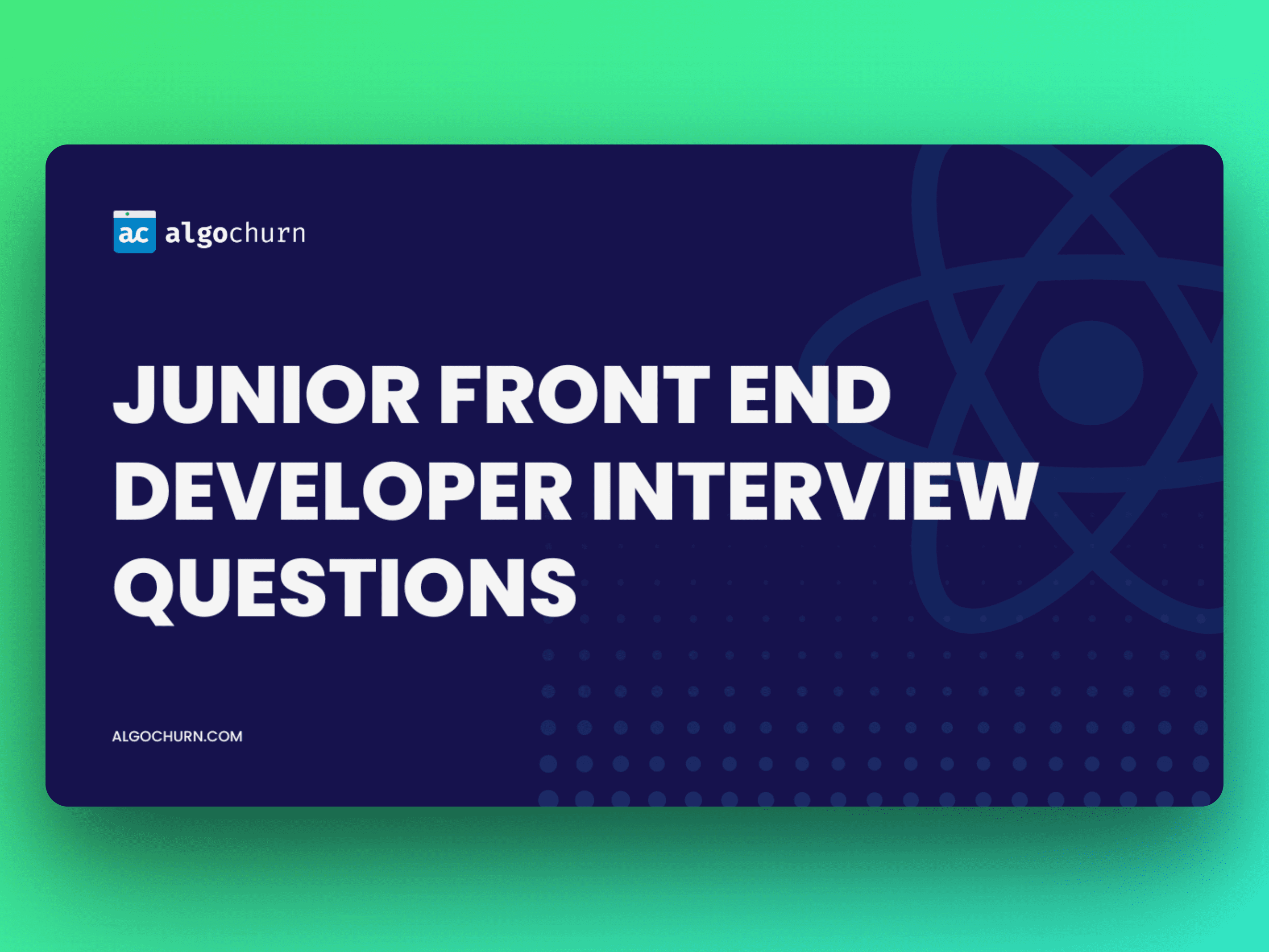
There are a lot of junior developer jobs out there. But what exactly do these positions entail? And what kind of questions should you expect if you're interviewing for one of these roles? As a junior front-end developer, your main responsibilities will be to assist senior developers with coding and troubleshooting tasks.
You'll also be responsible for creating and maintaining code documentation, as well as ensuring that all code meets industry standards. As far as interview questions go, expect to be asked about your coding experience and abilities, as well as your knowledge of HTML, CSS, and JavaScript. You may also be asked general questions about the software development process, so it's important to brush up on your terminology before the interview.
Array methods: what are they and how do you use them?
There are many different data structures that you can use to store collections of items, but arrays are perhaps the most popular and ubiquitous. An array is simply a sequence of elements, all of the same type, stored in contiguous memory locations. This makes accessing and manipulating the elements of an array very efficient. There are several different ways you can use arrays in your programs.
For example, you can use them to store lists of data, such as a list of students in a class or a list of products in a shopping cart. You can also use them to implement more sophisticated data structures like stacks and queues. And because they're so efficient, arrays are often used to hold large amounts of data that need to be processed quickly, such as searchable databases.
There are several methods that can be used to manipulate arrays, including adding, removing, and sorting items.
To use an array method, you must first find the method that you want to use and then call it on the array that you want to manipulate.
Some common array methods include .push()
, .pop()
, .sort()
, .map()
, .filter()
, .reduce()
.
If you want to practice, have a look at the top 5 front end interview questions asked in a tech interview round.
Javascript event loop: what is it and how does it work?
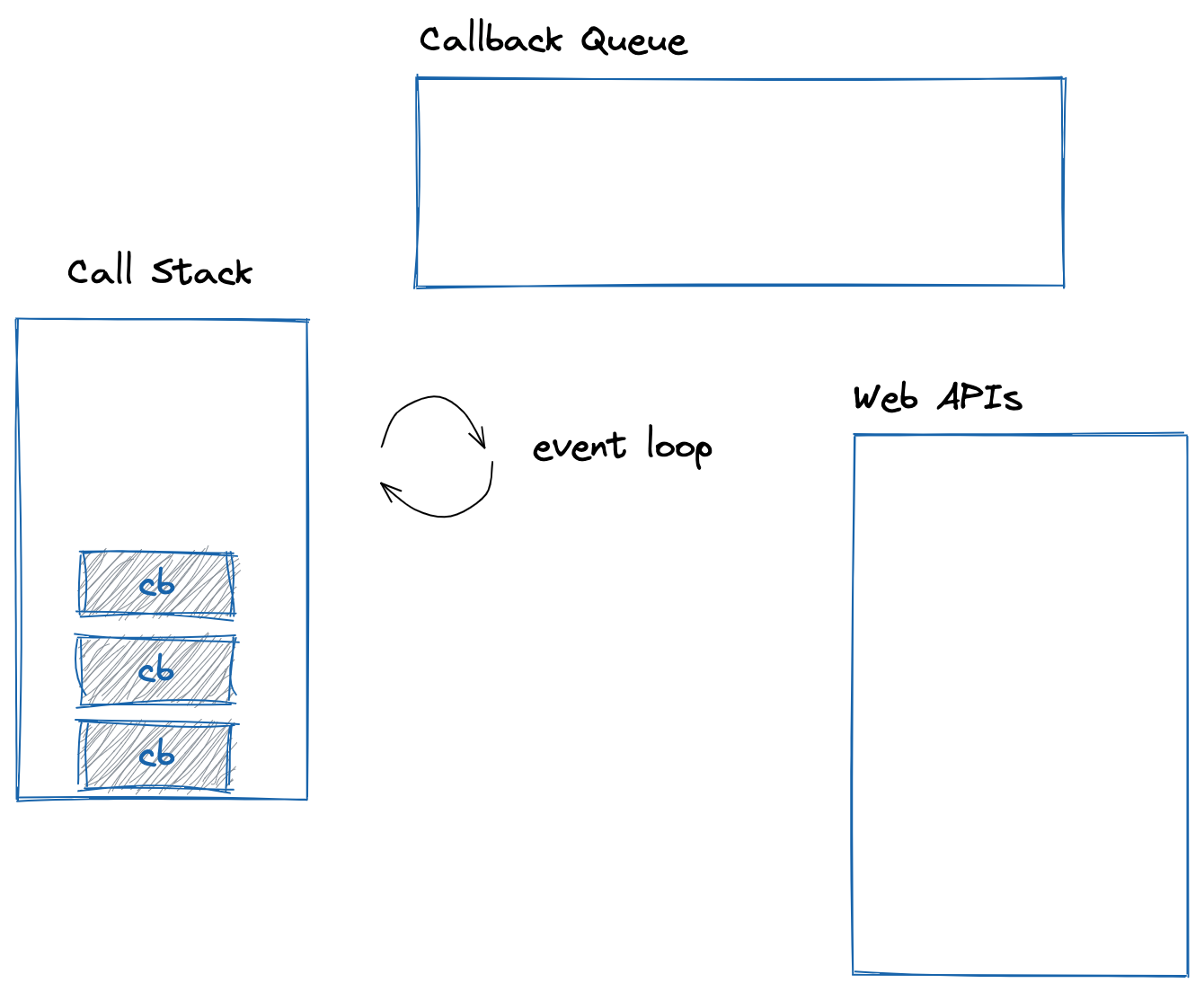
The Javascript event loop is a mechanism that allows for asynchronous code execution in Javascript.
The event loop works by continually checking the event queue for events that need to be processed, and then executing the corresponding code for those events.
Events can be anything from user input (such as clicking a button) to timer events (such as setTimeout()).
The event loop ensures that all events are processed in the correct order, and prevents race conditions from occurring.
Understanding the event loop is essential for understanding how asynchronous code works in Javascript, and is therefore an important topic for any junior front end developer to be familiar with.
The working of event loop is simple:
- It checks if the call stack is empty.
- If not, it takes the first item off the stack and runs it.
- Once the item has finished running, it checks for any callback functions that have been registered (such as event handlers), and adds them to the call stack.
- It then repeats the process until the call stack is empty.
Call, apply and bind in javascript: what are they and how do you use them?
There are three ways to set the this value in a function in javascript: call()
, apply()
and bind()
. Each of these functions takes the this value as its first argument. call()
and apply()
both invoke the function with the given this
value.
The difference between them is that call()
accepts additional arguments which are passed to the function, while apply()
accepts an array of arguments which are passed to the function. bind()
returns a new function with the given this
value set as its first argument.
When invoked, this new function will call the original function with the given this
value. You can use these functions to set the this
value explicitly, or to change how a function is called depending on what context it is being used in.
This keyword in javascript: what is it and how do you use it?
The this
keyword in JavaScript refers to the object that is currently being worked on. In other words, it gives you a way to access and manipulate the current object within a given context. You can use it to reference properties of the current object, invoke methods on the current object, etc.
You can use the this
keyword to access the properties and methods of an object.
The this
keyword in JavaScript is used to refer to the current object. In other words, it gives you a way to access the properties and methods of an object from inside that object. This can be particularly useful when you need to reference an object inside a method.
You can also use the this
keyword to call a method on an object.
When working with event handlers, the this
keyword can be used to reference the element that fired the event.
Example:
const person = {
firstName: "John",
lastName : "Doe",
id : 5566,
fullName : function() {
return this.firstName + " " + this.lastName;
}
};
Polyfills in javascript: what are they and why do you need them?
Polyfills are a type of javascript code that allows newer code to run on older browsers.
They are usually created by developers who want to use new features but need to support older browsers.
Polyfills can also be used to patch security holes or provide performance improvements on older browsers. They can also be used as a way to improve compatibility with newer web standards on older browsers. In some cases, polyfills can also provide fallbacks for features that are not yet supported by the browser.
When choosing a polyfill, it is important to consider the browser support you need as well as the size and performance of the code.
Some of the common polyfills problems are - .map()
, .filter()
, .reduce()
, Promise.all()
etc.
Practice how to create polyfills from scratch for the above mentioned methods.
React component lifecycle methods: what are they and how do you use them?
React component lifecycle methods allow you to control what happens when a component is created, updated, or destroyed.
You can use these methods to perform actions at specific points in a component's lifecycle, such as setting up timers or fetching data from an API.
The react-lifecycles-compat library provides compatibility with older versions of React, allowing you to use the latest lifecycle methods even if you're not using the latest React release.
If you're just starting out with React, it's important to understand how these methods work so that you can take full advantage of them in your components.
There are a few key things to keep in mind when working with React component lifecycle methods: make sure you understand the purpose of each method and when it should be invoked, don't try to access DOM nodes directly from within lifecycle methods (use refs instead), and be aware that changes made inside
The different component lifecycle methods in React are:-
componentWillMount()
: The componentWillMount
method is called before the component is rendered. This is a good place to set up an initial state or props that you want your component to have.
componentDidMount()
: This method is called when a component is first mounted, or rendered, on the screen. It can be used to set up any initial state for the component or to fetch data that the component needs to display. For example, if you need to load data from an external API in order to display it in your component, you would do that in this method.
componentWillReceiveProps()
: This method is called when a component is receiving new props. This will happen whenever the parent component to this one passes down new props. In this method, you can compare the current props (this.props) to the nextProps that will be received and perform some actions accordingly.
shouldComponentUpdate()
: The shouldComponentUpdate() method is invoked before the component is re-rendered, and it allows you to decide whether or not to update the component. This is useful if you want to optimize your component's performance by skipping unnecessary updates. To use this method, simply return false if you don't want the component to be updated.
For example:
shouldComponentUpdate(nextProps, nextState) {
return this.props.foo !== nextProps.foo;
}
componentWillUpdate()
: The componentWillUpdate method is invoked just before a component is updated. This method is not called for the initial render. Use this as an opportunity to perform last-minute preparations before the component is rendered. For example, you might want to check if a certain prop has changed and respond accordingly.
componentDidUpdate()
: The componentDidUpdate lifecycle method is invoked immediately after a component's updates are flushed to the DOM. This method is not called for the initial render.Use this lifecycle method to perform operations such as setting up a subscription or requesting data from an API, whenever a component's props or state changes. You can also access the previous props and state inside this method, if you need to compare them with the current values.
componentDidUpdate()
: The componentDidUpdate() method is invoked immediately after a component's updates are flushed to the DOM. This method is not called for the initial render.Use this as an opportunity to operate on the DOM when the component has been updated. This is also a good place to do network requests as long as you compare the current props with previous props (e.g. a fetch request may not be necessary if the data in state hasn't changed).
Each method serves a different purpose and is called at a different stage in the component's life cycle. For example, componentWillMount
is called before the component is rendered, while componentDidUpdate
is called after the component has been updated.
Debouncing in React: what is it and why is it important? 8. React hooks: what are they and how can you implement them in your code?
Debouncing in React is a technique for optimizing code performance by ensuring that a function is only executed once per set period of time. This can be particularly useful when working with complex or resource-intensive functions, such as those that make API calls or render large amounts of data. By debouncing a function, we can ensure that it's only executed once per interval, which can help to improve overall code performance.
There are a few reasons why debouncing is important in React:
- It can help improve performance by ensuring that costly operations (like re-rendering the UI) only happen once per event cycle, instead of on every user interaction.
- It can help prevent accidental duplicate submissions if a user clicks a button multiple times in quick succession.
- In some cases, like when fetching data from an API, debouncing can help ensure that we don't make too many requests in a short period of time and overwhelm the server.
Debouncing is a technique for rate-limiting function calls. It's often used to throttle expensive operations like API requests or rendering updates. In React, debouncing can be used to improve the performance of componentDidUpdate() by grouping multiple setState() calls into a single update.To implement debouncing in your code, you can use the lodash debounce function. This function takes two arguments: a function to call, and a delay in milliseconds. For example:
const debouncedFn = _.debounce(function () { // do something expensive here }, 1000);
This will call the given function once per second at most.
Alternatively, We have a question here on Algochurn that shows how to implement debouncing in a React application
Having an impressive GitHub profile helps a lot - here's how to make yours stand out!

As a junior front end developer, it's important to have an impressive GitHub profile. This is because your GitHub profile is one of the first things that potential employers will look at when considering you for a job.
Having a strong GitHub profile shows that you are active in the open source community and that you have the skills to work on real-world projects.
There are a few key things you can do to make your GitHub profile stand out. First, choose interesting and relevant projects to contribute to.
If you don't have any coding experience, consider contributing to documentation or design instead. Secondly, be sure to write clear and concise descriptions of your contributions so that employers can easily see what you did and how it benefited the project.
Finally, take advantage of social media platforms like Twitter and LinkedIn to promote your GitHub profile and show potential employers what you're working on.
If you're a junior front end developer, having an impressive GitHub profile can go a long way in helping you land a job. But what exactly makes a GitHub profile stand out?
Here are some tips:
-
Use a professional-looking profile photo. This can be a headshot or simply a picture that shows your face clearly.
-
Fill out your bio completely, including your location, education, and work experience (if any).
-
Choose an appropriate theme for your profile page. Something simple and clean is usually best.
-
Make sure all of your repositories are properly named and organized. This will help employers understand what you have worked on and how skilled you are in various programming languages and technologies.
-
If you have any open source contributions, highlight them on your profile page. This will show employers that you are active in the community and willing to share your work with others
Employers are looking for a few key things when they view a candidate's GitHub profile.
First, they want to see that the candidate is active on the platform and has a robust history of commits. They also want to see that the candidate is involved in various open source projects and has contributed significant code to those projects.
Finally, employers want to see that the candidate has a good understanding of version control systems and best practices. By demonstrating these qualities, candidates can make their GitHub profiles stand out from the rest.
Make sure you have a website to attract recruiters

As a junior front end developer, one of the best ways to attract recruiters and potential employers is to have an online portfolio.
A website is the perfect platform to showcase your skills, experience, and work samples. Plus, having a website shows that you are tech-savvy and capable of creating professional-looking web content.
If you don't already have a website, there's no need to worry - it's easy to create a simple, effective site that looks great.
All you need is a domain name and hosting, and you can use a drag-and-drop site builder or other tools to create your pages. Once your site is live, make sure to promote it on social media and in your email signature so potential employers can easily find it.
When it comes to creating a website that will attract recruiters, there are a few key elements you'll want to include.
First and foremost, your site should be well-designed and easy to navigate. Potential employers should be able to quickly find the information they're looking for, without getting lost in a maze of pages.
Secondly, your site should showcase your skills and experience in a clear and concise way. Include links to relevant projects you've worked on, as well as a professional headshot and bio.
Finally, make sure your contact information is readily available so recruiters can easily get in touch with you.
Here are some tips to make sure your website stands out when attracting recruiters:
-
Use an attention-grabbing design. Your website should have a clean and professional design, but it should also be visually interesting enough to catch a recruiter's eye.
-
Make sure your content is relevant and up-to-date. Recruiters will be looking for evidence that you have the skills and experience they're looking for, so make sure your website includes information on your latest projects and achievements.
-
Use social media to promote your website. Platforms like Twitter and LinkedIn are great ways to get your website in front of potential recruiters.